3rd Party Integrations
Any IoT system these days isn't complete without 3rd party integrations. With this in mind, we have added Alexa and Google Voice Assistant (GVA) integrations to ESP RainMaker.
Note: The standard examples in ESP RainMaker, viz. switch, led_light, fan and temperature_sensor are supported in Alexa/GVA. For a more exhaustive list, please check the docs here.
How does this work?
ESP RainMaker has a concept of devices and parameters and there are some standard types predefined for common use cases like switches and lightbulbs. We have now created a layer which maps these parameters to formats that are understood by Alexa and GVA. So a device type in RainMaker (like light, switch, etc.) maps to a similar device type there, and their parameters like power, brightness, hue, saturation, intensity. etc. get mapped to the corresponding capabilities/traits. If you have just the power and brightness params, you get a simple brightness controllable light. If you include hue, saturation and intensity, you get a color light in Alexa and GVA.
Usage
The code required to implement the standard devices is very simple. Let us look at a colour light example (led_light) which is already available on GitHub. The relevant code snippet below is self explanatory.
/* Create a standard lightbulb (internally adds name and power as mandatory parameters */
light_device = esp_rmaker_lightbulb_device_create("Light", NULL, DEFAULT_POWER);
esp_rmaker_device_add_cb(light_device, write_cb, NULL);
/* Add the standard brightness parameter */
esp_rmaker_device_add_param(light_device, esp_rmaker_brightness_param_create("brightness", DEFAULT_BRIGHTNESS));
/* Add the standard hue parameter */
esp_rmaker_device_add_param(light_device, esp_rmaker_hue_param_create("hue", DEFAULT_HUE));
/* Add the standard saturation parameter */
esp_rmaker_device_add_param(light_device, esp_rmaker_saturation_param_create("saturation", DEFAULT_SATURATION));
esp_rmaker_node_add_device(node, light_device);
The switch , fan and temperature sensor examples on GitHub are also ready out of the box for Alexa/GVA. Once you build and flash these examples, provision your board and link to your account using the ESP RainMaker Phone apps. Give the device some friendly name so that it is easy to identify.
Please also refer the section below if you want to build any custom devices.
Enabling Alexa
- Open the Alexa app on your phone, go to Skills and Games in the menu and search for "ESP RainMaker".
- Select the skill, tap on "Enable to Use" and provide your RainMaker credentials.
- Once the account linking is successful, allow Alexa to discover your devices.
- Once the devices are successfully discovered, the setup is complete and you can start controlling them using Alexa.
The latest ESP RainMaker phone apps also support enabling Alexa directly from the RainMaker app. Go to Settings -> Voice Services -> Amazon Alexa.
Enabling Google Voice Assistant (GVA)
- Open the Google Home app on your phone.
- Tap on "+" -> Set up Device.
- Select the "Works with Google" option meant for devices already set up.
- Search for ESP RainMaker and sign in using your RainMaker credentials.
- Once the Account linking is successful, your RainMaker devices will show up and you can start using them.
Building Custom Devices
Devices in RainMaker map to Display Categories in Alexa and Devices in GVA. Params in RainMaker map to Capabilities in Alexa and Traits in GVA. A list of devices and params that we currently support for Alexa and GVA can be found here. This list will go on increasing as and when we add support for more devices. You can also contact us if you want support for any of the standard devices.
Apart from the standard devices, Alexa can support custom devices too. Any device type, which is not supported in Alexa will show up as a device of type "Other" in Alexa. You can then add some standard parameters to it like esp.param.power
or use the generic controllers in Alexa, viz.
- Toggle controller : esp.param.toggle
- Mode controller : esp.param.mode
- Range controller : esp.param.range
Let us consider building an Air Cooler as an example, with following controls
- Power controller to on/off
- Toggle controller for swing on/off
- Range controller for Speed
- Mode controller for modes (Auto, Turbo, Sleep)
The code for that will look like this
/* Create an Air Cooler device and add the relevant parameters to it.
* You can choose any custom device type you want. Eg. "my.device.air-cooler"
*/
esp_rmaker_device_t *device = esp_rmaker_device_create("Air Cooler", "my.device.air-cooler", NULL);
esp_rmaker_device_add_cb(device, write_cb, NULL);
/* Standard Power Parameter */
esp_rmaker_param_t *primary = esp_rmaker_power_param_create("Power", true);
esp_rmaker_device_add_param(device, primary);
esp_rmaker_device_assign_primary_param(device, primary);
/* Generic Toggle Paramater */
esp_rmaker_param_t *swing = esp_rmaker_param_create("Swing", "esp.param.toggle", esp_rmaker_bool(true), PROP_FLAG_READ | PROP_FLAG_WRITE);
esp_rmaker_param_add_ui_type(swing, ESP_RMAKER_UI_TOGGLE);
esp_rmaker_device_add_param(device, swing);
/* Generic Range Parameter */
esp_rmaker_param_t *speed = esp_rmaker_param_create("Speed", "esp.param.range", esp_rmaker_int(3), PROP_FLAG_READ | PROP_FLAG_WRITE);
esp_rmaker_param_add_ui_type(speed, ESP_RMAKER_UI_SLIDER);
esp_rmaker_param_add_bounds(speed, esp_rmaker_int(0), esp_rmaker_int(5), esp_rmaker_int(1));
esp_rmaker_device_add_param(device, speed);
/* Generic Mode Parameter */
esp_rmaker_param_t *mode = esp_rmaker_param_create("Mode", "esp.param.mode", esp_rmaker_str("Auto"), PROP_FLAG_READ | PROP_FLAG_WRITE);
static const char *valid_strs[] = {"Auto", "Turbo", "Sleep"};
esp_rmaker_param_add_valid_str_list(mode, valid_strs, 3);
esp_rmaker_param_add_ui_type(mode, ESP_RMAKER_UI_DROPDOWN);
esp_rmaker_device_add_param(device, mode);
esp_rmaker_node_add_device(node, device);
Such a device, when discovered from Alexa will show up with these controls:
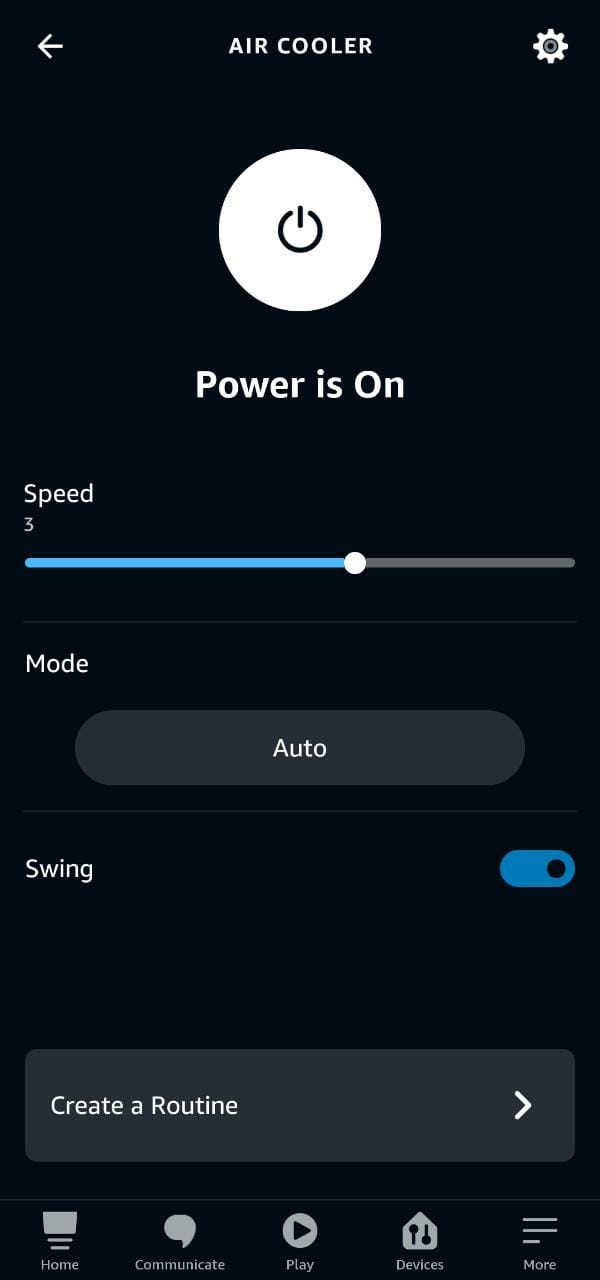
The same can also be controlled via Voice with invocations like
Alexa,
- Turn on the Air Cooler.
- Turn off the swing on Air Cooler.
- Set Air Cooler to Turbo mode.
- Set Air Cooler speed to 4.